Acure allows you to connect data from a wide variety of monitoring tools. However, events from primary systems often have complex names that do not help to simplify the analysis of the state of the infrastructure. Often a time for decision-making is in short supply, so you need to reduce the cognitive load and make the data understandable for perception.
Let’s look at how to do this in Acure using regular expressions and a new local function.
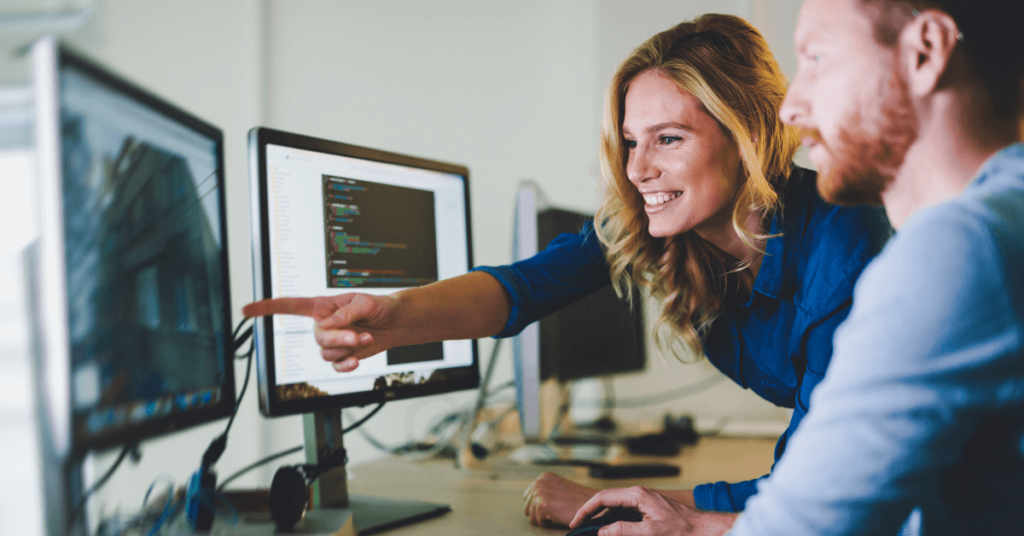
Event name conversion examples
💡 Imagine that you receive a problematic event from the primary monitoring system with the name SomeHost: High CPU utilization (over 90% for 5m).
For this event, we can apply the following regular expression “High.CPU.util.*over.(\\d+)%.*” and open the Signal in a readable form, with the name “CPU > 90%”.
💡 There can be a lot of examples of such transformations, for example, here is another one for a data storage system:
- Initial event name: “C:: Disk space is critically low (used > 90%)”
- Regular expression: “(.*): Disk space.*used.>.(.*)%.*”
- Resulting pattern: “$1 Storage Partition Usage > $2%”
- Signal Name: “C: Storage Partition Usage > 90%”
✅ We have implemented this request in the automation script as a local function that instantly converts values according to the dictionary defined in the same function. Unlike hard-coded global functions, local functions are a playground where you can implement any of your ideas within a C# script.
How event name conversion works in Acure
👉 To repeat such conversions in Acure, you need to create a local function in the Automation script and transform the Signal name before opening the signal.
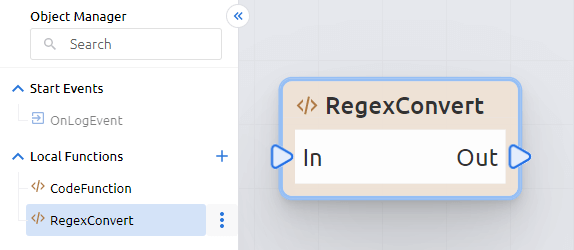
Function parameters:
🔵 Incoming pin – Input – a string containing the name of the event.
🔵 Outgoing pin – Result – a string containing the converted value.
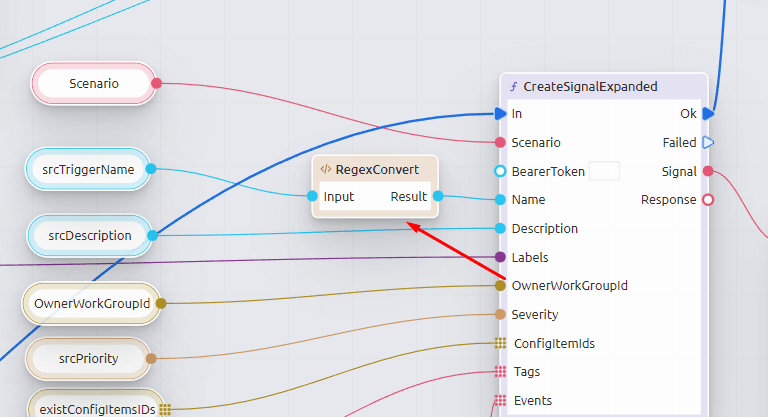
📜 Function code:
var regexDict = new Dictionary<string, string>()
{
{“(.*): Disk space.*used.>.(.*)%.*”,”$1: Storage partition usage > $2%”},
{“High memory util.*>(\\d+)%.*”,”RAM usage > $1%”},
{“High.CPU.util.*over.(\\d+)%.*”,”CPU Usage > $1%”},
};
foreach (var regex in regexDict)
{
if (!System.Text.RegularExpressions.Regex.IsMatch(Input, regex.Key))
continue;
return System.Text.RegularExpressions.Regex.Replace(Input, regex.Key, System.Text.RegularExpressions.Regex.Replace(regex.Value, “$”, “”));
}
returnInput;
***
⚙️ If the function finds a regular expression in its dictionary that matches the original event name, this value will be converted according to the pattern specified in the dictionary. If there is no matching regular expression in the dictionary, the original value will be returned.